Instructions
Instructions are statements that execute when we assemble the program. The instructions (written in assembly language) are translated into machine language bytes, as we have already discussed in the lecture 02. These machine language instructions/bytes are then executed by the CPU.
Example instruction: mov ax, bx
An assembly instruction has 4 basic parts:
- Label (optional)
- Mnemonic (required)
- Operand (depends on the instruction)
- Comment (optional)
Syntax of assembly language instruction is as follows:
[label:] mnemonic [operands] [;comment]
Note: We will be using Intel IA-32 for this tutorial and onwards.
Labels
There are two types of labels:
- Data Labels
- Code Labels
Data Label
Data labels are just like variables in any other language. For example, C language instruction: int myVar 10; we know that myVar is a variable name i.e. data label of int type containing value 10. Similarly, in assembly language we use data labels as variables which hold value (s) for us. For example, count is a variable in the following instruction:
count DWORD 100
Data labels are declared in data segment of the code.
Code Label
Code labels are just the names which are used by other instructions to jump from particular position to that position where data label is located. It must end with a semicolon ( : ). Code labels are declared in code segment. For example:
target:
mov ax, bx
...
jmp target
target is the data label, which is called by the jmp instruction.
Use: code labels are used as targets for jumping while using loops.
Mnemonics
A small word that acts as an identifier for the instruction. The mnemonics are written in code segment. In following examples mov, sub, add, jmp, call, and mul are the mnemonics:
- mov Move/assign one value to another (label)
- sub Subtract one value from another
- add Adds two values
- jmp Jump to a specific location
- call Call a procedure/module
- mul Multiply two values
Operands
Operand is the remaining part of the instruction. There can be 0 to 3 operands. Where each operand is either a memory operand, a register, an input/output port, or a constant expression.
Following are the operand types:
- constant expression
- constant
- memory (data label)
- register
Examples
- 0 operands
- stc ; set Carry flag
- 1 operand
- inc myByte ; memory
- inc eax ; register
- 2 operands
- sub myByte,25 ; memory, constant
- add ebx,ecx ; register, register
- add eax,36 * 25 ; register, constant-expression
Comments
You should have used comments in your programs before. As you know there are two types of comments: 1. Single-line and 2. Multi-line comments. In assembly single-line comments begin with ; (semicolon) whereas, multi-line comments begin with a comment directive and symbol/character selected by the programmer itself and also end with the same symbol/character.
Why you should use comments in your programs?
- explains the program's purpose
- tricky coding techniques
- revision information
- by whom and when it was written
- application-specific explanations
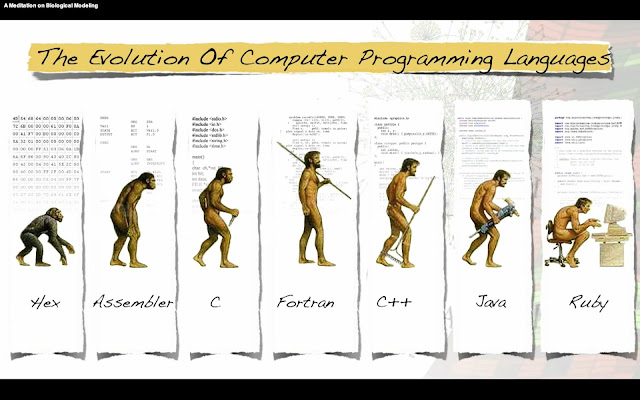
dsadasdasdadfqfaaaaasdfhnfdtjdfSv vcSYKbg vf
ReplyDelete